Let's forget frameworks and libraries for a moment. Today we’re just going to talk about the <form />
element and some of the things you can do with it in the DOM.
For web devs who are getting a little long in the tooth, you’re probably familiar with most of this, but for newer devs, this might be news to you. Either way, buckle up because we’re about to go old school with forms.
With newer DOM APIs like querySelector
and querySelectorAll
, we can access forms via a selector, e.g. document.querySelector(‘form’);
. Did you know that you can also access forms directly from the document
? There is an HTMLCollections
of forms available via document.forms
. Go ahead, I’ll let you open the dev tools in your favourite editor. Pretty neat eh?
So let’s say we’re on amazon.ca.
You have a form that looks like this:
<form class="nav-searchbar" name="site-search">
...
</form>
OK, so you know there is a document.forms
object. Let’s take a peek in the dev tools.
document.forms[0]
finds our form, and there is one other form on the page, but there’s also two more properties on document.forms
. There’s site-search
and ue_backdetect
. If we look at the markup above for our form, we see it has a name
attribute with the value ’site-search'
. That’s one of the extra properties on document.forms
. Sure enough, document.forms[‘site-search’]
gives us a form. If we do document.forms[0] === document.forms[‘site-search’]
in the console of our dev tools, we’ll see that it returns true.
If you haven’t caught on yet, this means that you can access forms via an index, which represents the order they appear in the DOM, but you can also access it via a form’s name
attribute.
Alright, there’s more folks. Let’s get a reference to our form by running the following in the console, const siteSearchForm = document.forms['site-search']
.
Now let’s see what properties are available on our form.
OK, so we see some properties like action
, for the URL to GET
/POST
to, but as we go down the list, there’s one called elements
. Hmm, what could that be? 😉 If we access it in the dev tools console, we get the following:
What? All the form inputs are in there! That’s right folks, you have access to all the <input />
s, <textarea />
s etc via this property. Pretty cool eh? Not only that, if the form inputs have a name
attribute, we can do the same thing we did for document.forms
. We can access the form inputs by index or their name, e.g. siteSearchForm.elements[‘field-keywords’]
.
So accessing forms and form inputs can be done right off the document
via the document.forms
property.
One last fun fact. Each form element has a form
property which is a reference to the <form />
DOM node that the form element is contained within.
Hope you enjoyed this DOM Throwback Thursday.
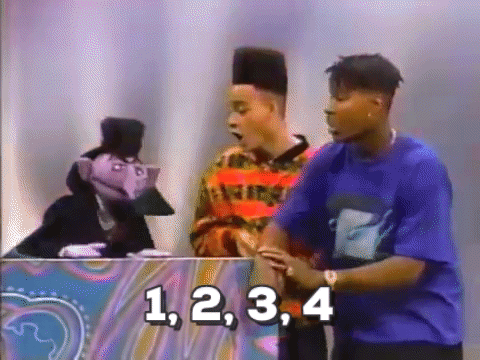